[백준 1890 : JAVA] 점프 / DP + DFS
문제
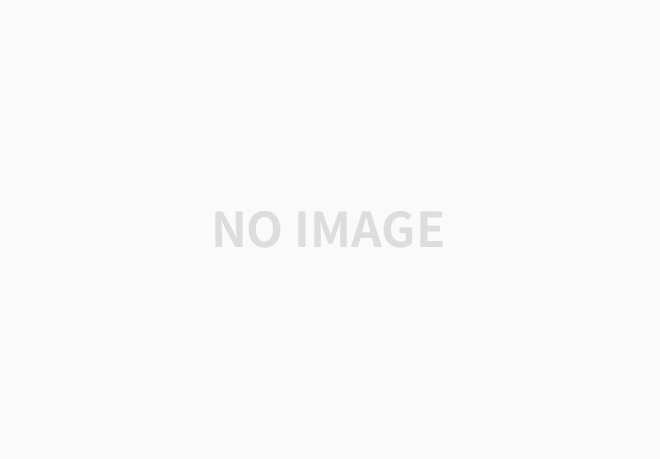
풀이
BFS로 문제를 풀어보려고 고민을 여러 차례해봤는데, BFS로는 탐색했던 곳을 재탐색하지 않으면서, 경로의 수를 누적시키는게 불가능하다고 판단했다. 반면, DFS를 이용하면 하나의 경로에 대해 온전히 보장받으면서 재탐색없이 경로의 수를 누적시키는게 가능하다.
아래의 그림을 참고하자.
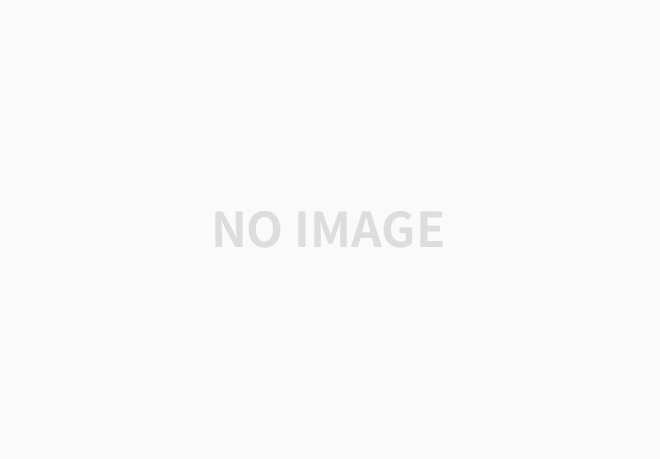
즉, 도착점까지 탐색을 진행한 후에, 역으로 거슬러 올라오면서 경로의 값을 누적시키는게 포인트다.
코드
import java.io.*; import java.util.*; public class Main { private static int N; private static int[][] map; private static long[][] cache; private static int[] dx = {1, 0}; private static int[] dy = {0, 1}; public static void main(String[] args) throws Exception { BufferedReader br = new BufferedReader(new InputStreamReader(System.in)); N = Integer.parseInt(br.readLine()); cache = new long[N + 1][N + 1]; map = new int[N + 1][N + 1]; for (int i = 1; i <= N; i++) { StringTokenizer st = new StringTokenizer(br.readLine()); for (int j = 1; j <= N; j++) { map[i][j] = Integer.parseInt(st.nextToken()); } } dfs(1, 1); System.out.println(cache[1][1]); } public static boolean dfs(int x, int y) { if (map[y][x] == 0) { if (x == N && y == N) { cache[N][N] = 1; return true; } return false; } boolean isSearchFinish = false; // 해당 노드를 기준으로 오른쪽, 아래쪽의 탐색을 마쳤는지 체크. for (int i = 0; i < dx.length; i++) { int nx = (dx[i] == 0 ? x : x + map[y][x]); int ny = (dy[i] == 0 ? y : y + map[y][x]); if (!isValidRange(nx, ny)) continue; // 다음 지점에서 이미 탐색이 진행되었다면 if (cache[ny][nx] > 0) { cache[y][x] += cache[ny][nx]; // 현재 지점에서 도착지까지 이동할 수 있는 경우의 수 추가하기 isSearchFinish = true; continue; } if (dfs(nx, ny)) { cache[y][x] += cache[ny][nx]; isSearchFinish = true; } } return isSearchFinish; } private static boolean isValidRange(int x, int y) { return (1 <= x && x <= N) && (1 <= y && y <= N); } }
'Algorithm > Baekjoon' 카테고리의 다른 글
[백준 2302 : JAVA] 극장 좌석 / DP (2) | 2021.04.18 |
---|---|
[백준 10164 : JAVA] 격자상의 경로 / DFS + DP (0) | 2021.04.17 |
[백준 2225 : JAVA] 합분해 / DP (0) | 2021.04.08 |
[백준 2133 : JAVA] 타일 채우기 / DP (0) | 2021.04.06 |
[백준 7469 : JAVA] K번째 수 / MergeSort Tree (0) | 2021.04.02 |
댓글
이 글 공유하기
다른 글
-
[백준 2302 : JAVA] 극장 좌석 / DP
[백준 2302 : JAVA] 극장 좌석 / DP
2021.04.18 -
[백준 10164 : JAVA] 격자상의 경로 / DFS + DP
[백준 10164 : JAVA] 격자상의 경로 / DFS + DP
2021.04.17 -
[백준 2225 : JAVA] 합분해 / DP
[백준 2225 : JAVA] 합분해 / DP
2021.04.08 -
[백준 2133 : JAVA] 타일 채우기 / DP
[백준 2133 : JAVA] 타일 채우기 / DP
2021.04.06
댓글을 사용할 수 없습니다.